In part 1, we got started by introducing an application where digital identity is stored in a blockchain, focusing on the use case and the proposed system architecture. In this part, we will cover the implementation details and key takeaways.
Implementation
Modern and distributed applications require a modern and distributed data platform for streamlined development and rapid scale as the network grows. Storing the data locally is not reliable, performant or scalable enough, and here is where the advantages of the MongoDB Atlas cloud database and the MongoDB Stitch serverless platform really shine, providing the best foundation to build apps with a global reach.
MongoDB Stitch
MongoDB Stitch, MongoDB’s serverless platform, allowed us to utilize several major capabilities to improve our development speed, security, and scale. Here’s how:
- Authentication models: Various authentication and user management features allow us to easily authenticate decentralized nodes in the blockchain network (using anonymous authentication). On the other hand, consumers of the network would authenticate with advanced security mechanisms to secure their data access. For simplicity our example uses email/password authentication:

async function handleLogin() {
 const email = loginEmailEl.value;
 const password = loginPasswordEl.value;
 const credential = new UserPasswordCredential(email, password);
 
 try {
 
 await stitchClient.auth.loginWithCredential(credential);
 const user = stitchClient.auth.user;
 showLoggedInState();
 displaySuccess(`Logged in as: ${user.profile.data.email}`)

 } catch(e) {
 handleError(e)
 }
}

- Stitch Rules: Flexible and easy to define authorization rules that can be applied on collection, field, and document levels give us the ability to manage data access in very sophisticated and controlled ways. One of the most fascinating abilities is to automatically project fields such as “credit_score” and “assets_range” only if a user has permissions to view them. Stitch roles allow you to filter data based on a user’s preferences:

"assets_range": {
 "read": {
 "%or": [
 {
 "%%root.owner_id": "%%user.id"
 },
 { "%%root.identity_verification_log.transaction_details.assets_shared": "assets_range"
 }
 ]
 }
 }

- Stitch Functions: Implement hosted, server-side logic that can govern process accuracy and validate data used within the data access rules. For example,
approvedByMajority
verification will return true when the block actually has a majority of votes from participating blockchain nodes:
function(block){
 // Get collections 
 var nodes = context.services.get("mongodb-atlas").db("assets").collection("nodes");
 var pending_blocks = context.services.get("mongodb-atlas").db("transactions").collection("pending_blocks");
 
 // Retrieve the current block
 var doc = pending_blocks.findOne({index : block.index});
 if (doc)
 {
 // Retrieve approvals vs active nodes
 currentApprovals = doc.approvals;
 
 var numberOfActiveNodes = nodes.count( {"active" : true});
 var approvedNodes = nodes.count({"owner_id" : {"$in" : currentApprovals}});
 
 // See if majority has approved
 if (approvedNodes > (numberOfActiveNodes / 2))
 {
 return true;
 }
 else
 {
 return false;
 }
 
 }
 return false;
};

- Stitch Triggers & third-party services: Once a user’s identity has been validated, Stitch Triggers we notify parties about offers and promotions based on the data in the blockchain.
With third-party service integrations, we can integrate information and security gathering services easily into the process.
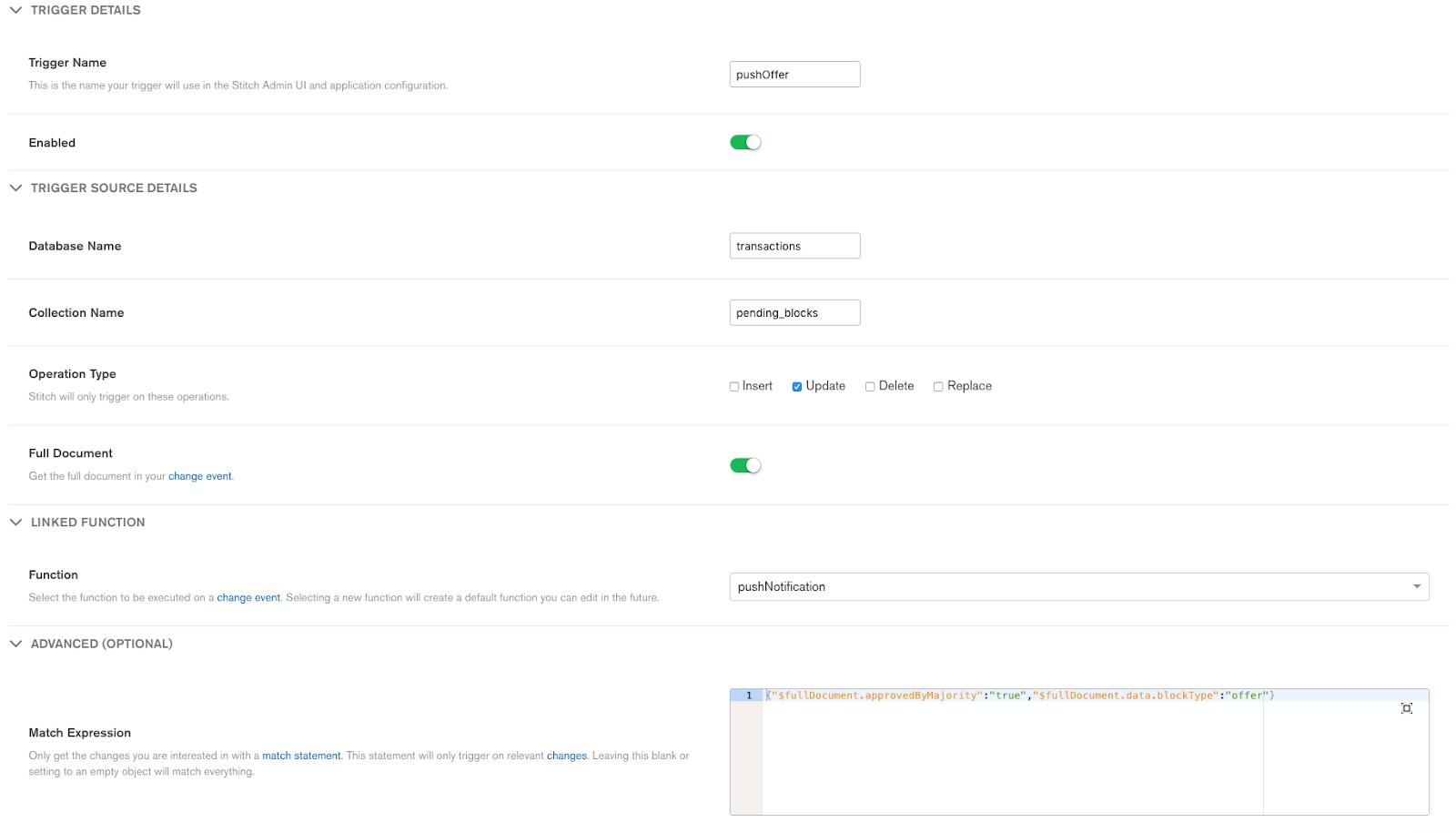
MongoDB Atlas
MongoDB Stitch is backed with an Atlas cluster which provides us with 4 key abilities:
- Atlas provides data access and management with scalability, resilience, and worldwide distribution to conform with privacy regulations such as GDPR and HIPAA.
- Rich query and analytical language with a built-in Stitch hybrid connection string. In particular, we have utilized MongoDB views built on top of the $graphLookup aggregation stage, which is a key capability to traverse and validate data structures such as Blockchains.

db.createView("blockchain","pending_blocks",
 [ {
 "$match" : {
 "index" : 0
 }
 },
 {
 "$graphLookup" : {
 "from" : "pending_blocks",
 "startWith" : "$previousHash",
 "connectFromField" : "hash",
 "connectToField" : "previousHash",
 "as" : "chain"
 }
 },
 {
 "$unwind" : "$chain"
 },
 {
 "$sort" : {
 "chain.index" : 1
 }
 },
 {
 "$group" : {
 "_id" : "$_id",
 "chain" : {
 "$push" : "$chain"
 }
 }
 },
 {
 "$project" : {
 "chain" : 1,
 "_id" : 0
 }
 }
 ]);

db.pending_blocks.createIndex({index : 1},{unique : true});


- Change Streams features are a tremendous game changer for event-driven applications. Any data changing events can be filtered within Atlas when only relevant notifications to the application watcher. One main use case for this is when nodes receive a notification about a new block generated by one of the network workers:

// Configure change pipeline
const pipeline = [
 { $match: {'fullDocument.owner_id' : { "$ne" : client.authedId() },
 "operationType" : "insert" } }
 ];
 // Get collection and set a change stream
 var db = atlasConn.db('transactions');
 const changeStream = db.collection('pending_blocks').watch(pipeline);

- Built-in TLS and enterprise security features allow us to enforce additional levels of access control, auditing, and encryption, layering upon the governance features of MongoDB Stitch and the Blockchain itself.
Conclusions & Key Takeaways
The world of blockchain in digital systems has a tremendous potential and I believe we are going to see some extremely innovative ideas that will thrive outside of the cryptocurrency space. MongoDB enables rapid innovation, developer productivity, and scale-out for applications like this one and many others – which all benefit from decentralized data control, trust and immutability.
- MongoDB 18 Talk: Decentralized Identity Management with Blockchain and MongoDB
- White Paper: Building Enterprise Grade Blockchain Databases with MongoDB.